Adicionando nova coluna ao DataFrame existente no Pandas
Vamos discutir como adicionar novas colunas ao DataFrame existente no Pandas. Existem várias maneiras de fazermos essa tarefa.
Método # 1: declarando uma nova lista como uma coluna.
# Import pandas package
import pandas as pd
# Define a dictionary containing Students data
data = {'Name': ['Jai', 'Princi', 'Gaurav', 'Anuj'],
'Height': [5.1, 6.2, 5.1, 5.2],
'Qualification': ['Msc', 'MA', 'Msc', 'Msc']}
# Convert the dictionary into DataFrame
df = pd.DataFrame(data)
# Declare a list that is to be converted into a column
address = ['Delhi', 'Bangalore', 'Chennai', 'Patna']
# Using 'Address' as the column name
# and equating it to the list
df['Address'] = address
# Observe the result
df
Saída:
Observe que o comprimento da sua lista deve corresponder ao comprimento da coluna do índice, caso contrário, ele mostrará um erro.
<iframe allow="accelerometer; autoplay; clipboard-write; encrypted-media; gyroscope; picture-in-picture" allowfullscreen="" frameborder="0" height="374" src="https://www.youtube.com/embed/IZ8oCcVB3iA?feature=oembed" width="665"></iframe>
Método 2: usando DataFrame.insert()
Dá a liberdade de adicionar uma coluna em qualquer posição que quisermos e não apenas no final. Ele também fornece opções diferentes para inserir os valores da coluna.
# Import pandas package
import pandas as pd
# Define a dictionary containing Students data
data = {'Name': ['Jai', 'Princi', 'Gaurav', 'Anuj'],
'Height': [5.1, 6.2, 5.1, 5.2],
'Qualification': ['Msc', 'MA', 'Msc', 'Msc']}
# Convert the dictionary into DataFrame
df = pd.DataFrame(data)
# Using DataFrame.insert() to add a column
df.insert(2, "Age", [21, 23, 24, 21], True)
# Observe the result
df
Saída:
Método 3: Usando o método Dataframe.assign()
Este método criará um novo dataframe com uma nova coluna adicionada ao antigo dataframe.
# Import pandas package
import pandas as pd
# Define a dictionary containing Students data
data = {'Name': ['Jai', 'Princi', 'Gaurav', 'Anuj'],
'Height': [5.1, 6.2, 5.1, 5.2],
'Qualification': ['Msc', 'MA', 'Msc', 'Msc']}
# Convert the dictionary into DataFrame
df = pd.DataFrame(data)
# Using 'Address' as the column name and equating it to the list
df2 = df.assign(address = ['Delhi', 'Bangalore', 'Chennai', 'Patna'])
# Observe the result
df2
Resultado:
Método 4: Usando um dicionário
Podemos usar um dicionário Python para adicionar uma nova coluna no pandas DataFrame. Use uma coluna existente como os valores-chave e seus respectivos valores serão os valores da nova coluna.
# Import pandas package
import pandas as pd
# Define a dictionary containing Students data
data = {'Name': ['Jai', 'Princi', 'Gaurav', 'Anuj'],
'Height': [5.1, 6.2, 5.1, 5.2],
'Qualification': ['Msc', 'MA', 'Msc', 'Msc']}
# Define a dictionary with key values of
# an existing column and their respective
# value pairs as the # values for our new column.
address = {'Delhi': 'Jai', 'Bangalore': 'Princi',
'Patna': 'Gaurav', 'Chennai': 'Anuj'}
# Convert the dictionary into DataFrame
df = pd.DataFrame(data)
# Provide 'Address' as the column name
df['Address'] = address
# Observe the output
df
Saída:
As postagens do blog Acervo Lima te ajudaram? Nos ajude a manter o blog no ar!
Faça uma doação para manter o blog funcionando.
70% das doações são no valor de R$ 5,00...
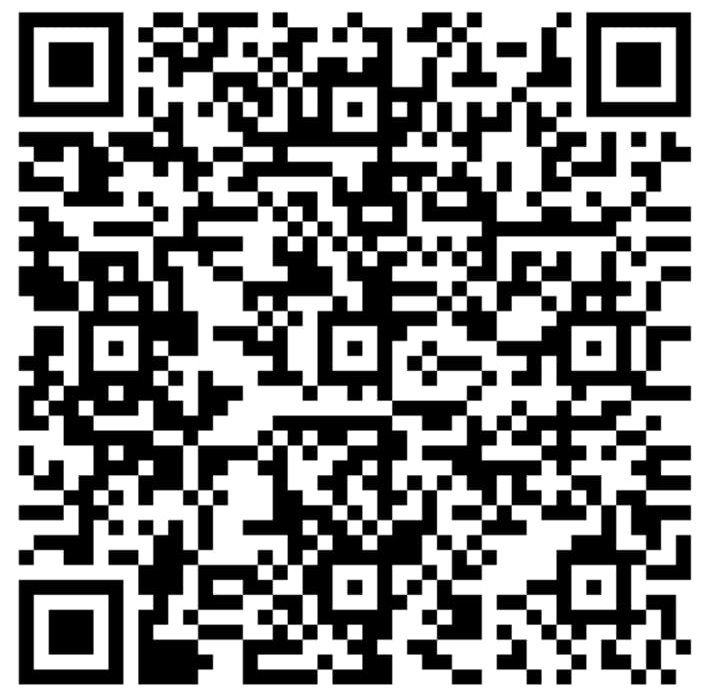
Diógenes Lima da Silva
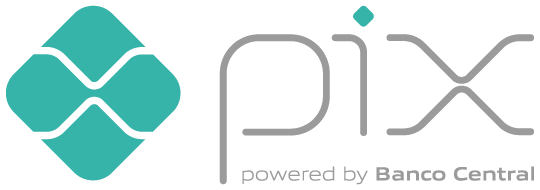