Como obter uma caixa de diálogo se não houver conexão com a Internet usando jQuery?
Neste artigo, veremos como verificar a conexão com a Internet usando jQuery. Estaremos usando navigator.onLine, que retornará verdadeiro se houver uma conexão com a Internet disponível, caso contrário, retornará falso .
Sintaxe:
navigator.onLine
Retorna:
- Verdadeiro: se a conexão com a Internet estiver disponível.
- Falso: se a conexão com a Internet não estiver disponível.
Exemplo 1: Este exemplo irá verificar se a conexão com a Internet está disponível ou não e exibir uma caixa de alerta ao clicar no botão.
<!DOCTYPE html>
<html>
<head>
<!--Including JQuery-->
<script src=
"https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script>
$(document).ready(function () {
// Function to be called on button click
$("button").click(function () {
// Detecting the internet connection
var online = navigator.onLine;
if (online) {
// Showing alert when connection is available
$("#message").show().html("Connected!");
} else {
// Showing alert when connection is not available
alert("No connection available");
}
});
});
</script>
</head>
<body>
<p>
Click the button below to check
your internet connection.
</p>
<button>Check connection</button>
<div style="height: 10px"></div>
<div id="message"></div>
</body>
</html>
Saída:
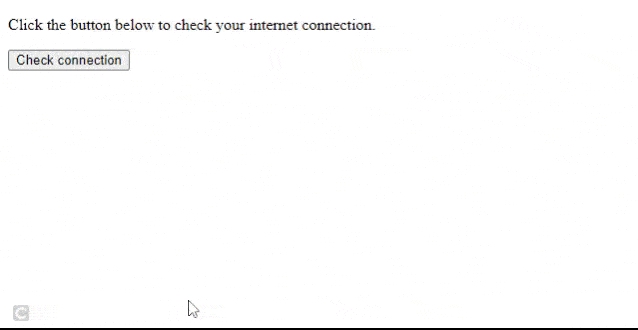
Verifique a conexão
Exemplo 2 : Este exemplo verifica automaticamente se há uma conexão com a Internet a cada 3 segundos. Se não houver conexão com a Internet, ele mostrará um alerta.
<!DOCTYPE html>
<html>
<head>
<!--Including JQuery-->
<script src=
"https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js">
</script>
<script>
// Function to check internet connection
function checkInternetConnection() {
// Detecting the internet connection
var online = navigator.onLine;
if (!online) {
// Showing alert when connection is not available
$("#message").show().html("No connection available");
}
}
// Setting interval to 3 seconds
setInterval(checkInternetConnection, 3000);
</script>
</head>
<body>
<p>
It will automatically check for internet
connection after every 3 seconds.
</p>
<div style="height: 10px"></div>
<div id="message"></div>
</body>
</html>
Saída:
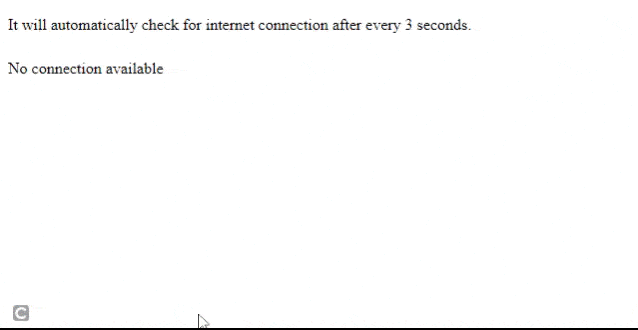
Sem conexão disponível
As postagens do blog Acervo Lima te ajudaram? Nos ajude a manter o blog no ar!
Faça uma doação para manter o blog funcionando.
70% das doações são no valor de R$ 5,00...
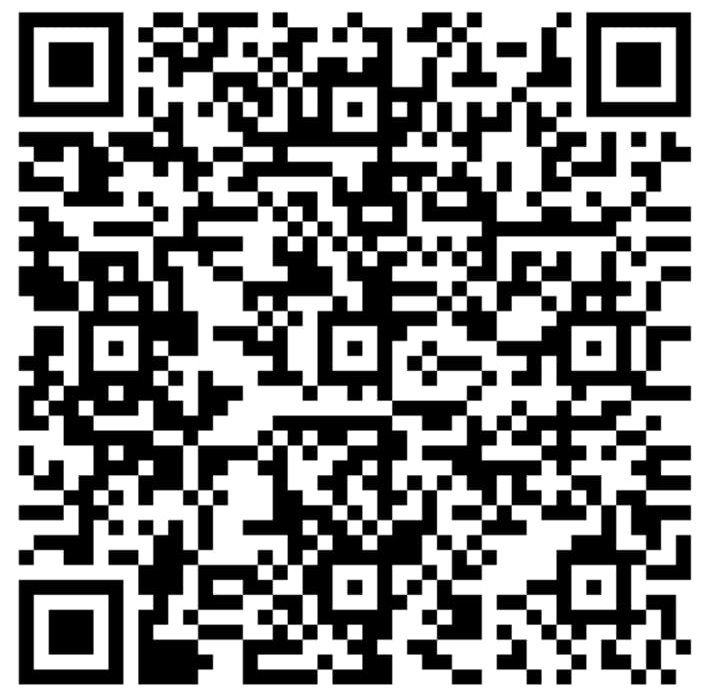
Diógenes Lima da Silva
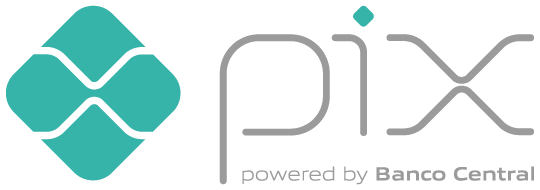