Como usar a expressão regular como substituto do método endsWith() em Java?
Portanto, discuta principalmente o que é o método endsWith(), portanto, é um método da classe String que verifica se a string termina com um sufixo especificado. Este método retorna um valor booleano verdadeiro ou falso.
Sintaxe:
public boolean endsWith(String suff)
Parâmetro: parte do sufixo especificado
Retorno: valor booleano, aqui em java temos apenas verdadeiro e falso.
Métodos:
Podemos usar expressões regulares / correspondências como um substituto para o método startWith() em Java
- Usando Expressão Regular
- Usando fósforos
Método 1: usando expressões regulares
Expressões regulares ou Regex (em resumo) é uma API para definir padrões de String que podem ser usados para pesquisar, manipular e editar uma string em Java. Validação de e-mail e senhas são algumas áreas de strings em que o Regex é amplamente usado para definir as restrições. Expressões regulares são fornecidas no pacote java.util.regex. Este consiste em 3 classes e 1 interface. O pacote java.util.regex consiste principalmente nas três classes a seguir, conforme descrito abaixo em formato tabular, da seguinte maneira:
- Padrão
- Matcher
- PatternSyntaxException
Exemplo
// Java Program to Illustrate use of Regular Expression
// as substitute of endsWith() method
// Importing required classes
import java.util.regex.Matcher;
import java.util.regex.Pattern;
// Main class
public class Geek {
// Main driver method
public static void main(String args[])
{
// Declaring and initialising string
String Str = new String("Welcome to geeksforgeeks");
// Display message
System.out.print(
"Check whether string ends with Welcome using endsWith : ");
// Using endWith() method
System.out.println(Str.endsWith("geeks"));
// Creating Pattern and matcher classes object
Pattern pattern = Pattern.compile(".*geeks");
Matcher m = pattern.matcher(Str);
// Checking whether string ends with specific word
// or nor and returning the boolean value
// using ? operator and find() method
System.out.print(
"Check whether string ends with Welcome using Regex: ");
System.out.println(m.find() ? "true" : "false");
}
}
Verifique se a string termina com Welcome usando endsWith: true Verifique se a string termina com Welcome using Regex: true
Método 2: usando correspondências
O método String.matches() informa se esta string corresponde ou não à expressão regular fornecida. Uma invocação desse método da forma str.matches (regex) produz exatamente o mesmo resultado que a expressão Pattern.matches (regex, str).
Sintaxe:
public boolean matches(String regex);
Parâmetros: a expressão regular com a qual esta string deve ser correspondida.
Valor de retorno: este método retorna verdadeiro se, e somente se, essa string corresponder à expressão regular fornecida.
Exemplo
// Java Program to Illustrate use of Matches
// as substitute of endsWith() method
// Importing all utility classes
import java.util.*;
// Main class
public class Geek {
// Main driver method
public static void main(String args[])
{
// Initialising String
String Str = new String("Welcome to geeksforgeeks");
// Display message for better readibility
System.out.print(
"Check whether string starts with Welcome using endsWith : ");
// Using endsWith() to end with specific word
System.out.println(Str.endsWith("geeks"));
// Display message for better readibility
System.out.print(
"Check whether string starts with Welcome using Matches: ");
// Now checking whether it matches with
// above defined specific word
System.out.println(Str.matches("(.*)geeks"));
}
}
Verifique se a string começa com Welcome usando endsWith: true Verifique se a string começa com Welcome using Matches: true
As postagens do blog Acervo Lima te ajudaram? Nos ajude a manter o blog no ar!
Faça uma doação para manter o blog funcionando.
70% das doações são no valor de R$ 5,00...
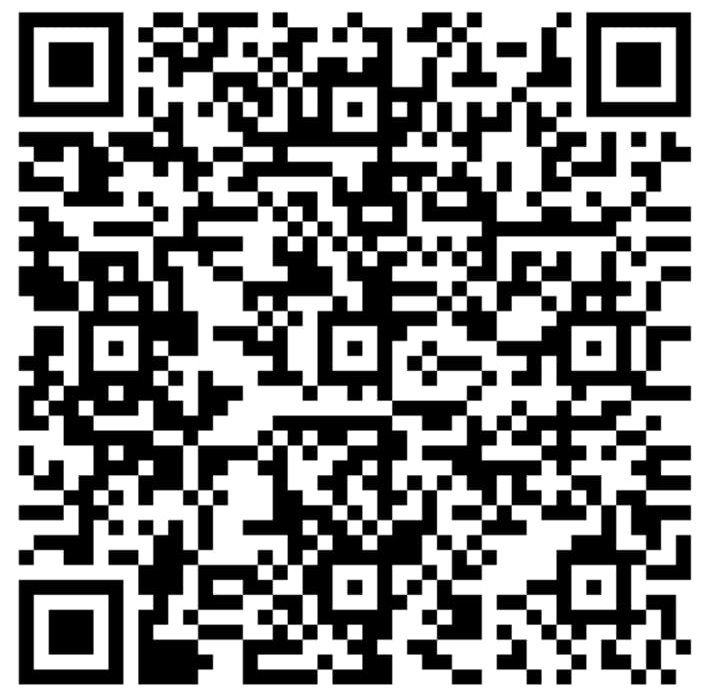
Diógenes Lima da Silva
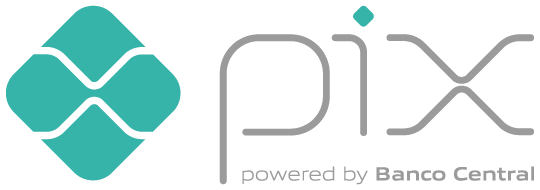