Desenhe um quadrado e um retângulo em tartaruga - Python
Pré-requisito: Turtle Programming Basics
turtle é um módulo embutido em Python. Oferece desenho em tela (cartolina) e tartaruga (caneta). Para desenhar algo na tela, precisamos mover a tartaruga (caneta). Para mover a tartaruga, existem algumas funções, ou seja, para a frente(), para trás(), etc.
Desenho Quadrado:
import
turtle
t
=
turtle.Turtle()
s
=
int
(
input
(
"Enter the length of the side of the Squre: "
))
t.forward(s)
t.left(
90
)
t.forward(s)
t.left(
90
)
t.forward(s)
t.left(
90
)
t.forward(s)
t.left(
90
)
Entrada :
100
Resultado :
Segunda abordagem (usando Loop):
import
turtle
t
=
turtle.Turtle()
s
=
int
(
input
(
"Enter the length of the side of squre: "
))
for
_
in
range
(
4
):
t.forward(s)
t.left(
90
)
Entrada :
100
Resultado :
Retângulo de desenho:
import
turtle
t
=
turtle.Turtle()
l
=
int
(
input
(
"Enter the length of the Rectangle: "
))
w
=
int
(
input
(
"Enter the width of the Rectangle: "
))
t.forward(l)
t.left(
90
)
t.forward(w)
t.left(
90
)
t.forward(l)
t.left(
90
)
t.forward(w)
t.left(
90
)
Entrada :
100 120
Resultado :
Segunda abordagem (usando Loop):
import
turtle
t
=
turtle.Turtle()
l
=
int
(
input
(
"Enter the length of the Rectangle: "
))
w
=
int
(
input
(
"Enter the width of the Rectangle: "
))
for
_
in
range
(
4
):
if
_
%
2
=
=
0
:
t.forward(l)
t.left(
90
)
else
:
t.forward(w)
t.left(
90
)
Entrada :
100 120
Resultado :
Desde agora, você deve ter aprendido a desenhar várias ilustrações geométricas básicas como círculo, quadrado, retângulo. Então, vamos implementar esse conhecimento para construir algo que você possa realmente usar na construção de jogos como vamos desenhar um ser humano usando o conhecimento básico do conhecimento geométrico.
Aqui está o código para esta implementação: -
import
turtle
def
draw_dream():
window
=
turtle.Screen()
window.bgcolor(
"white"
)
draw_Scarlett()
window.exitonclick()
def
draw_Scarlett():
brad
=
turtle.Turtle()
brad.shape(
"turtle"
)
brad.color(
"red"
)
draw_head(brad)
draw_body(brad)
draw_arm(brad)
draw_leg1(brad)
draw_leg2(brad)
def
draw_head(brad):
brad.circle(
60
)
brad.speed(
3
)
brad.right(
60
)
def
draw_body(brad):
num
=
0
while
num <
3
:
brad.forward(
150
)
brad.right(
120
)
brad.speed(
1
)
num
+
=
1
def
draw_arm(brad):
brad.forward(
60
)
brad.left(
60
)
brad.forward(
60
)
brad.backward(
60
)
brad.right(
60
)
brad.backward(
60
)
brad.right(
60
)
brad.forward(
60
)
brad.right(
60
)
brad.forward(
60
)
brad.backward(
60
)
brad.left(
60
)
brad.forward(
90
)
def
draw_leg1(brad):
brad.left(
120
)
brad.forward(
40
)
brad.right(
120
)
brad.forward(
120
)
draw_foot1(brad)
def
draw_leg2(brad):
brad.color(
"red"
)
brad.right(
180
)
brad.forward(
120
)
brad.right(
60
)
brad.forward(
70
)
brad.right(
60
)
brad.forward(
120
)
draw_foot2(brad)
def
draw_foot1(brad):
brad.color(
"blue"
)
num
=
0
while
num <
4
:
brad.forward(
20
)
brad.right(
90
)
num
+
=
1
def
draw_foot2(brad):
brad.color(
"blue"
)
num
=
0
while
num <
4
:
brad.forward(
20
)
brad.left(
90
)
num
+
=
1
draw_dream()
Resultado:
As postagens do blog Acervo Lima te ajudaram? Nos ajude a manter o blog no ar!
Faça uma doação para manter o blog funcionando.
70% das doações são no valor de R$ 5,00...
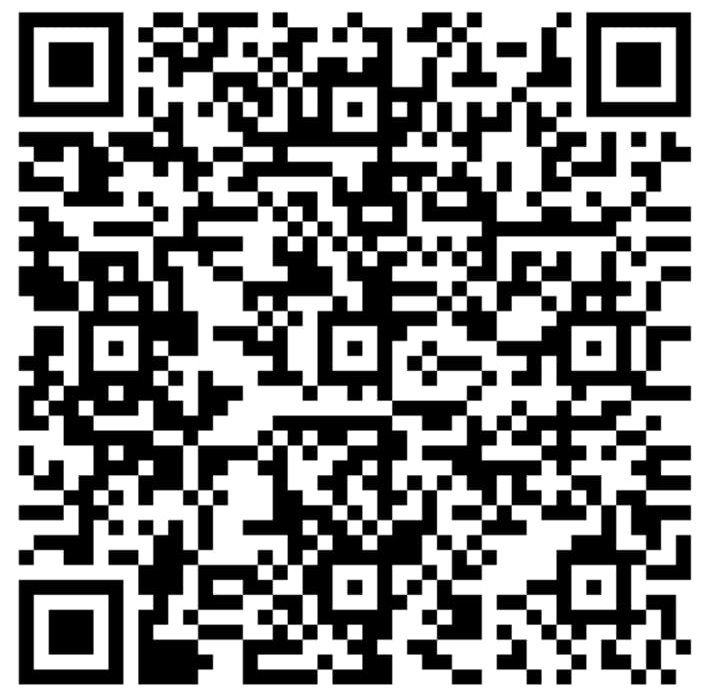
Diógenes Lima da Silva
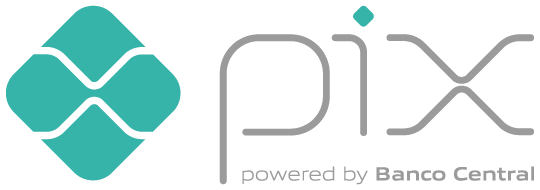