Função deflater setInput() em Java com exemplos
A função setInput() da classe Deflater em java.util.zip é usada para definir dados de entrada para compactação. Esta função deve ser chamada quando needsInput() retorna true, indicando que o buffer de entrada está vazio.
Assinatura da função:
public void setInput(byte[] b) public void setInput(byte[] b, int offset, int len)
Sintaxe:
d.setInput(byte[]); d.setInput(byte[], int, int);
Parâmetro: os vários parâmetros aceitos por essas funções sobrecarregadas são:
- byte [] b : Esta é a matriz de entrada que deve ser esvaziada
- deslocamento interno : Este é o deslocamento inicial a partir do qual os valores devem ser lidos na matriz fornecida
- comprimento interno : Este é o comprimento máximo a ser compactado a partir do deslocamento inicial.
Tipo de retorno: A função não retorna nada.
Exceção: a função não lança nenhuma exceção
Exemplo 1:
// Java program to describe the use
// of setInput(byte[] b) function
import java.util.zip.*;
import java.io.UnsupportedEncodingException;
class GFG {
public static void main(String args[])
throws UnsupportedEncodingException
{
// deflater
Deflater d = new Deflater();
// get the text
String pattern = "GeeksforGeeks", text = "";
// generate the text
for (int i = 0; i < 4; i++)
text += pattern;
// set the Input for deflator
d.setInput(text.getBytes("UTF-8"));
// finish
d.finish();
// output bytes
byte output[] = new byte[1024];
// compress the data
int size = d.deflate(output);
// compressed String
System.out.println("Compressed String :"
+ new String(output)
+ "\n Size " + size);
// original String
System.out.println("Original String :" + text
+ "\n Size " + text.length());
// end
d.end();
}
}
Saída:
Compressed String :x?sOM?.N?/r???q?? Size 21 Original String :GeeksforGeeksGeeksforGeeksGeeksforGeeksGeeksforGeeks Size 52
Exemplo 2:
// Java program to describe the use
// of setInput(byte[] b, int offset, int len) function
import java.util.zip.*;
import java.io.UnsupportedEncodingException;
class GFG {
public static void main(String args[])
throws UnsupportedEncodingException
{
// deflater
Deflater d = new Deflater();
// get the text
String pattern = "GeeksforGeeks", text = "";
// generate the text
for (int i = 0; i < 4; i++)
text += pattern;
// set the Input for deflator
d.setInput(text.getBytes("UTF-8"), 10, 30);
// finish
d.finish();
// output bytes
byte output[] = new byte[1024];
// compress the data
int size = d.deflate(output);
// compressed String
System.out.println("Compressed String :"
+ new String(output)
+ "\n Size " + size);
// end
d.end();
}
}
Saída:
Compressed String :x?K?.vOM?.N?/???? Size 22
Referência: https://docs.oracle.com/javase/7/docs/api/java/util/zip/Deflater.html#setInput()
As postagens do blog Acervo Lima te ajudaram? Nos ajude a manter o blog no ar!
Faça uma doação para manter o blog funcionando.
70% das doações são no valor de R$ 5,00...
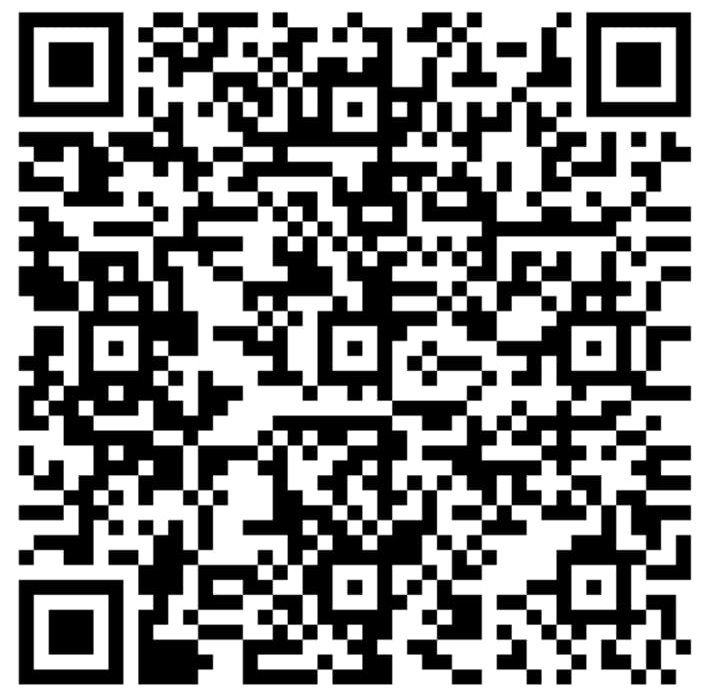
Diógenes Lima da Silva
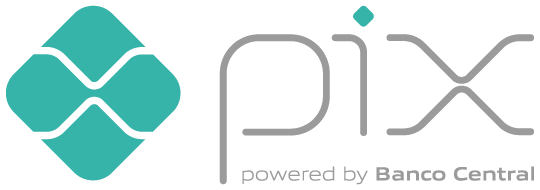