Método Hashtable containsKey() em Java
O método java.util.Hashtable.containsKey() é usado para verificar se uma determinada chave está presente na Hashtable ou não. Ele pega o elemento chave como parâmetro e retorna True se esse elemento estiver presente na tabela.
Sintaxe:
Hash_table.containsKey(key_element)
Parâmetros: O método usa apenas um parâmetro key_element que se refere à chave cuja presença deve ser verificada dentro de uma Hashtable.
Valor de retorno: o método retorna verdadeiro booleano se a chave estiver presente na tabela de hash, caso contrário, ele retorna falso.
Os programas abaixo são usados para ilustrar o funcionamento do Método java.util.Hashtable.containsKey():
Programa 1:
// Java code to illustrate the containsKey() method
import java.util.*;
public class Hash_Table_Demo {
public static void main(String[] args)
{
// Creating an empty Hashtable
Hashtable<Integer, String> hash_table =
new Hashtable<Integer, String>();
// Putting values into the table
hash_table.put(10, "Geeks");
hash_table.put(15, "4");
hash_table.put(20, "Geeks");
hash_table.put(25, "Welcomes");
hash_table.put(30, "You");
// Displaying the Hashtable
System.out.println("Initial Table is: " + hash_table);
// Checking for the key_element '20'
System.out.println("Is the key '20' present? " +
hash_table.containsKey(20));
// Checking for the key_element '5'
System.out.println("Is the key '5' present? " +
hash_table.containsKey(5));
}
}
A tabela inicial é: {10 = Geeks, 20 = Geeks, 30 = You, 15 = 4, 25 = Welcome} A chave '20' está presente? verdade A chave '5' está presente? falso
Programa 2:
// Java code to illustrate the containsKey() method
import java.util.*;
public class Hash_Table_Demo {
public static void main(String[] args)
{
// Creating an empty Hashtable
Hashtable<String, Integer> hash_table=
new Hashtable<String, Integer>();
// Putting values into the table
hash_table.put("Geeks", 10);
hash_table.put("4", 15);
hash_table.put("Geeks", 20);
hash_table.put("Welcomes", 25);
hash_table.put("You", 30);
// Displaying the Hashtable
System.out.println("Initial Table is: " + hash_table);
// Checking for the key_element 'Welcomes'
System.out.println("Is the key 'Welcomes' present? " +
hash_table.containsKey("Welcomes"));
// Checking for the key_element 'World'
System.out.println("Is the key 'World' present? " +
hash_table.containsKey("World"));
}
}
A tabela inicial é: {You = 30, Welcome = 25, 4 = 15, Geeks = 20} A chave 'Boas-vindas' está presente? verdade A chave 'Mundo' está presente? falso
Nota: A mesma operação pode ser realizada com qualquer tipo de variação e combinação de diferentes tipos de dados.
As postagens do blog Acervo Lima te ajudaram? Nos ajude a manter o blog no ar!
Faça uma doação para manter o blog funcionando.
70% das doações são no valor de R$ 5,00...
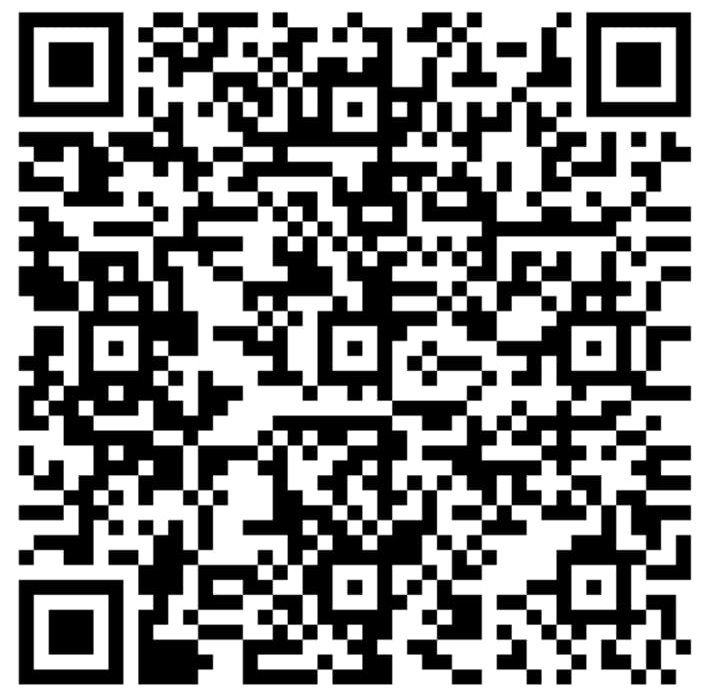
Diógenes Lima da Silva
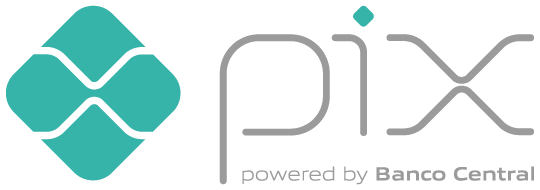