Método keys() em Hashtable em Java
O método java.util.Hashtable.keys() da classe Hashtable em Java é usado para obter a enumeração das chaves presentes na tabela de hash.
Sintaxe:
Enumeration enu = Hash_table.keys()
Parâmetros: o método não aceita nenhum parâmetro.
Valor de retorno: o método retorna uma enumeração das chaves do Hashtable.
Os programas abaixo são usados para ilustrar o funcionamento do método java.util.Hashtable.keys():
Programa 1:
// Java code to illustrate the keys() method
import java.util.*;
public class Hash_Table_Demo {
public static void main(String[] args)
{
// Creating an empty Hashtable
Hashtable<Integer, String> hash_table =
new Hashtable<Integer, String>();
// Inserting elements into the table
hash_table.put(10, "Geeks");
hash_table.put(15, "4");
hash_table.put(20, "Geeks");
hash_table.put(25, "Welcomes");
hash_table.put(30, "You");
// Displaying the Hashtable
System.out.println("The Table is: " + hash_table);
// Creating an empty enumeration to store
Enumeration enu = hash_table.keys();
System.out.println("The enumeration of keys are:");
// Displaying the Enumeration
while (enu.hasMoreElements()) {
System.out.println(enu.nextElement());
}
}
}
Saída:
A mesa é: {10 = Geeks, 20 = Geeks, 30 = You, 15 = 4, 25 = Welcome} A enumeração das chaves são: 10 20 30 15 25
Programa 2:
// Java code to illustrate the keys() method
import java.util.*;
public class Hash_Table_Demo {
public static void main(String[] args)
{
// Creating an empty Hashtable
Hashtable<String, Integer> hash_table =
new Hashtable<String, Integer>();
// Inserting elements into the table
hash_table.put("Geeks", 10);
hash_table.put("4", 15);
hash_table.put("Geeks", 20);
hash_table.put("Welcomes", 25);
hash_table.put("You", 30);
// Displaying the Hashtable
System.out.println("The Table is: " + hash_table);
// Creating an empty enumeration to store
Enumeration enu = hash_table.keys();
System.out.println("The enumeration of keys are:");
// Displaying the Enumeration
while (enu.hasMoreElements()) {
System.out.println(enu.nextElement());
}
}
}
Saída:
A tabela é: {You = 30, Welcome = 25, 4 = 15, Geeks = 20} A enumeração das chaves são: Vocês Boas-vindas 4 Geeks
As postagens do blog Acervo Lima te ajudaram? Nos ajude a manter o blog no ar!
Faça uma doação para manter o blog funcionando.
70% das doações são no valor de R$ 5,00...
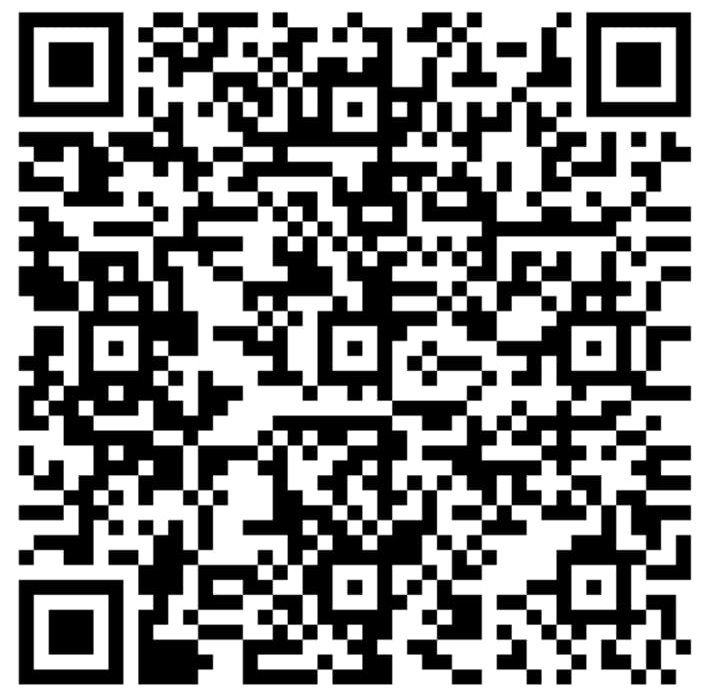
Diógenes Lima da Silva
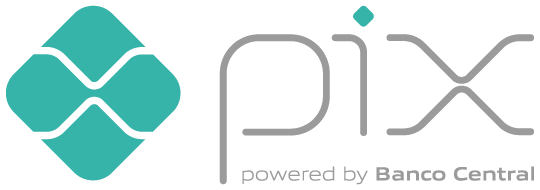