Método Split() String em Java com exemplos
O método string split() quebra uma determinada string em torno das correspondências da expressão regular fornecida.
Por exemplo:
Input String: 016-78967 Regular Expression: - Output : {"016", "78967"}
A seguir estão as duas variantes do método split() em Java:
1. Divisão de String pública [] (string regex, limite interno)
Parameters: regex - a delimiting regular expression Limit - the result threshold Returns: An array of strings computed by splitting the given string. Throws: PatternSyntaxException - if the provided regular expression’s syntax is invalid.
O parâmetro de limite pode ter 3 valores:
limit > 0 : If this is the case then the pattern will be applied at most limit-1 times, the resulting array’s length will not be more than n, and the resulting array’s last entry will contain all input beyond the last matched pattern. limit < 0 : In this case, the pattern will be applied as many times as possible, and the resulting array can be of any size. limit = 0 : In this case, the pattern will be applied as many times as possible, the resulting array can be of any size, and trailing empty strings will be discarded.
Funciona assim:
Let the string to be splitted be : geekss@for@geekss Regex Limit Result @ 2 {“geekss”, ”for@geekss”} @ 5 {“geekss”, ”for”, ”geekss”} @ -2 {“geekss”, ”for”, ”geekss”} s 5 {“geek”, ”“, “@for@geek”, “”, “”} s -2 {“geek”, ” “, “@for@geek”, “”, “”} s 0 {“geek”, ””, ”@for@geek”}
A seguir estão os códigos de exemplo Java para demonstrar o funcionamento de split()
Exemplo 1:
// Java program to demonstrate working of split(regex,
// limit) with small limit.
public class GFG {
public static void main(String args[])
{
String str = "geekss@for@geekss";
String[] arrOfStr = str.split("@", 2);
for (String a : arrOfStr)
System.out.println(a);
}
}
Saída:
geekss for@geekss
Exemplo 2:
// Java program to demonstrate working of split(regex,
// limit) with high limit.
public class GFG {
public static void main(String args[])
{
String str = "geekss@for@geekss";
String[] arrOfStr = str.split("@", 5);
for (String a : arrOfStr)
System.out.println(a);
}
}
Saída:
geekss for geekss
Exemplo 3:
// Java program to demonstrate working of split(regex,
// limit) with negative limit.
public class GFG {
public static void main(String args[])
{
String str = "geekss@for@geekss";
String[] arrOfStr = str.split("@", -2);
for (String a : arrOfStr)
System.out.println(a);
}
}
Saída:
geekss for geekss
Exemplo 4:
// Java program to demonstrate working of split(regex,
// limit) with high limit.
public class GFG {
public static void main(String args[])
{
String str = "geekss@for@geekss";
String[] arrOfStr = str.split("s", 5);
for (String a : arrOfStr)
System.out.println(a);
}
}
Saída:
geek @for@geek
Exemplo 5:
// Java program to demonstrate working of split(regex,
// limit) with negative limit.
public class GFG {
public static void main(String args[])
{
String str = "geekss@for@geekss";
String[] arrOfStr = str.split("s", -2);
for (String a : arrOfStr)
System.out.println(a);
}
}
Saída:
geek @for@geek
Exemplo 6:
// Java program to demonstrate working of split(regex,
// limit) with 0 limit.
public class GFG {
public static void main(String args[])
{
String str = "geekss@for@geekss";
String[] arrOfStr = str.split("s", 0);
for (String a : arrOfStr)
System.out.println(a);
}
}
Saída:
Geek @for@geek
2. public String [] split (String regex)
Esta variante do método de divisão usa uma expressão regular como parâmetro e quebra a string fornecida em torno das correspondências desta expressão regular regex. Aqui, por padrão, o limite é 0.
Parameters: regex - a delimiting regular expression Returns: An array of strings computed by splitting the given string. Throws: PatternSyntaxException - if the provided regular expression’s syntax is invalid.
Aqui estão alguns exemplos de códigos de trabalho:
Exemplo 1:
// Java program to demonstrate working of split()
public class GFG {
public static void main(String args[])
{
String str = "GeeksforGeeks:A Computer Science Portal";
String[] arrOfStr = str.split(":");
for (String a : arrOfStr)
System.out.println(a);
}
}
Saída:
GeeksforGeeks A Computer Science Portal
Exemplo 2:
// Java program to demonstrate working of split()
public class GFG {
public static void main(String args[])
{
String str = "GeeksforGeeksforStudents";
String[] arrOfStr = str.split("for");
for (String a : arrOfStr)
System.out.println(a);
}
}
Saída:
Geeks Geeks Students
Pode ser visto no exemplo acima que o padrão / expressão regular “para” é aplicada duas vezes (porque “para” está presente duas vezes na string a ser dividida)
Exemplo 3:
// Java program to demonstrate working of split()
public class GFG {
public static void main(String args[])
{
String str = "Geeks for Geeks";
String[] arrOfStr = str.split(" ");
for (String a : arrOfStr)
System.out.println(a);
}
}
Saída:
Geeks for Geeks
Exemplo 4:
// Java program to demonstrate working of split()
public class GFG {
public static void main(String args[])
{
String str = "Geekssss";
String[] arrOfStr = str.split("s");
for (String a : arrOfStr)
System.out.println(a);
}
}
Saída:
Geek
No exemplo acima, essas strings vazias não estão incluídas no array resultante arrOfStr.
Exemplo 5:
// Java program to demonstrate working of split()
public class GFG {
public static void main(String args[])
{
String str = "GeeksforforGeeksfor ";
String[] arrOfStr = str.split("for");
for (String a : arrOfStr)
System.out.println(a);
}
}
Saída:
geeks geeks
No exemplo acima, os espaços finais (portanto, não uma string vazia) no final se tornam uma string no array resultante arrOfStr.
Exemplo 6:
// Java program to demonstrate working of split()
// using regular expressions
public class GFG {
public static void main(String args[])
{
String str = "word1, word2 word3@word4?word5.word6";
String[] arrOfStr = str.split("[, ?.@]+");
for (String a : arrOfStr)
System.out.println(a);
}
}
word1 word2 word3 word4 word5 word6
No exemplo acima, as palavras são separadas sempre que um dos caracteres especificados no conjunto é encontrado.
Este artigo é uma contribuição de Vaibhav Bajpai . Se você gosta de GeeksforGeeks e gostaria de contribuir, você também pode escrever um artigo usando contribute.geeksforgeeks.org ou enviar o seu artigo para contribute@geeksforgeeks.org. Veja o seu artigo na página principal do GeeksforGeeks e ajude outros Geeks.
Escreva comentários se encontrar algo incorreto ou se quiser compartilhar mais informações sobre o tópico discutido acima.
As postagens do blog Acervo Lima te ajudaram? Nos ajude a manter o blog no ar!
Faça uma doação para manter o blog funcionando.
70% das doações são no valor de R$ 5,00...
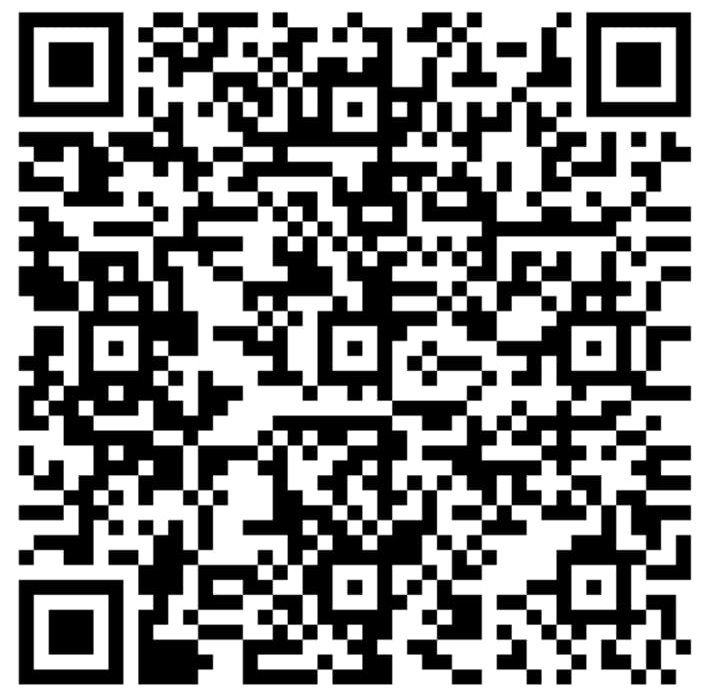
Diógenes Lima da Silva
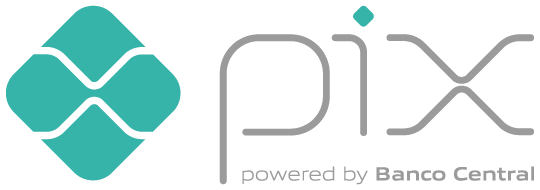