Python PostgreSQL - Ordenar por
Neste artigo, discutiremos como usar a cláusula order by no PostgreSQL usando python.
A cláusula Order By é usada para classificar os registros de uma tabela retornada pela cláusula SELECT em ordem crescente por padrão, mas a palavra-chave asc pode ser usada. Se quisermos classificar os registros em ordem decrescente, temos que escrever a palavra desc .
Sintaxe:
SELECT column1, column2, .... FROM table_name ORDER BY column1, colum2,.... [ASC | DESC]
Dados em uso:
Para começar, primeiro importe todas as bibliotecas necessárias para o espaço de trabalho e, em seguida, estabeleça a conexão com o banco de dados. Agora inicialize um cursor e passe a instrução SQL a ser executada. Imprima o conjunto de resultados gerado e feche a conexão.
Exemplo 1: código Python para exibir o nome do estado em ordem decrescente
# importing psycopg2 module
import psycopg2
# establishing the connection
conn = psycopg2.connect(
database="postgres",
user='postgres',
password='password',
host='localhost',
port='5432'
)
# creating cursor object
cursor = conn.cursor()
# creating table
sql = '''CREATE TABLE Geeks(
id SERIAL NOT NULL,
name varchar(20) not null,
state varchar(20) not null
)'''
cursor.execute(sql)
# inserting values in the table
cursor.execute('''INSERT INTO Geeks(name , state) VALUES ('Babita','Bihar')''')
cursor.execute(
'''INSERT INTO Geeks(name , state) VALUES ('Anushka','Hyderabad')''')
cursor.execute(
'''INSERT INTO Geeks(name , state) VALUES ('Anamika','Banglore')''')
cursor.execute('''INSERT INTO Geeks(name , state) VALUES ('Sanaya','Pune')''')
cursor.execute(
'''INSERT INTO Geeks(name , state) VALUES ('Radha','Chandigarh')''')
# query to sort table by descending order of state
sql2 = 'select * from Geeks order by state desc;'
# executing query
cursor.execute(sql2)
# fetching records
print(cursor.fetchall())
# Commit your changes in the database
conn.commit()
# Closing the connection
conn.close()
Saída:
[(4, 'Sanaya', 'Pune'), (2, 'Anushka', 'Hyderabad'), (5, 'Radha', 'Chandigarh'), (1, 'Babita', 'Bihar'), ( 3, 'Anamika', 'Banglore')]
Exemplo 2: código Python para exibir registros de Geeks em ordem crescente de nome
# importing psycopg2 module
import psycopg2
# establishing the connection
conn = psycopg2.connect(
database="postgres",
user='postgres',
password='password',
host='localhost',
port='5432'
)
# creating cursor object
cursor = conn.cursor()
# creating table
sql = '''CREATE TABLE Geeks(
id SERIAL NOT NULL,
name varchar(20) not null,
state varchar(20) not null
)'''
cursor.execute(sql)
# inserting values in the table
cursor.execute('''INSERT INTO Geeks(name , state) VALUES ('Babita','Bihar')''')
cursor.execute(
'''INSERT INTO Geeks(name , state) VALUES ('Anushka','Hyderabad')''')
cursor.execute(
'''INSERT INTO Geeks(name , state) VALUES ('Anamika','Banglore')''')
cursor.execute('''INSERT INTO Geeks(name , state) VALUES ('Sanaya','Pune')''')
cursor.execute(
'''INSERT INTO Geeks(name , state) VALUES ('Radha','Chandigarh')''')
# query to sort table by name
sql2 = 'select * from Geeks order by name;'
# executing query
cursor.execute(sql2)
# fetching records
print(cursor.fetchall())
# Commit your changes in the database
conn.commit()
# Closing the connection
conn.close()
Saída:
[(3, 'Anamika', 'Banglore'), (2, 'Anushka', 'Hyderabad'), (1, 'Babita', 'Bihar'), (5, 'Radha', 'Chandigarh'), ( 4, 'Sanaya', 'Pune')]
Atenção geek! Fortaleça suas bases com o Python Programming Foundation Course e aprenda o básico.
As postagens do blog Acervo Lima te ajudaram? Nos ajude a manter o blog no ar!
Faça uma doação para manter o blog funcionando.
70% das doações são no valor de R$ 5,00...
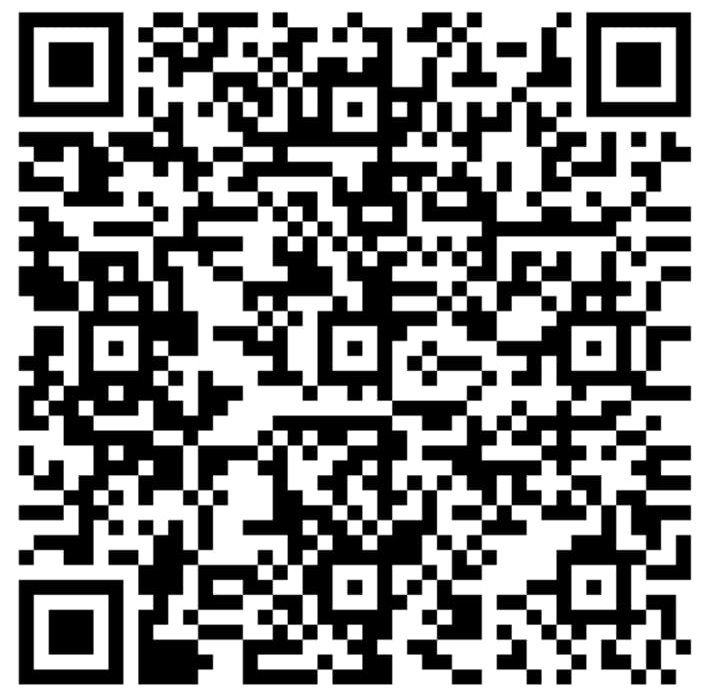
Diógenes Lima da Silva
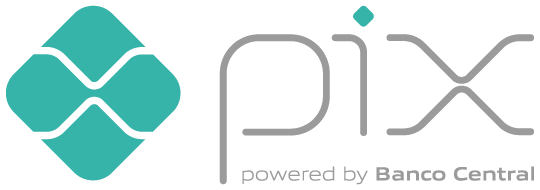