Quebra-cabeça de queda de ovo | DP-11
A seguir está uma descrição da instância deste famoso quebra-cabeça envolvendo n = 2 ovos e um edifício com k = 36 andares.
Suponha que desejamos saber quais histórias em um prédio de 36 andares são seguras para derrubar ovos e quais farão com que os ovos se quebrem na aterrissagem. Fazemos algumas suposições:
... Um ovo que sobrevive a uma queda pode ser usado novamente.
… ..Um ovo quebrado deve ser descartado.
… ..O efeito de uma queda é o mesmo para todos os ovos.
… ..Se um ovo quebrar ao cair, ele quebraria se cair de um andar superior.
... Se um ovo sobreviver a uma queda, ele sobreviverá a uma queda mais curta.
… ..Não está descartado que as janelas do primeiro andar quebrem ovos, nem está descartado que o 36º andar não cause a quebra de um ovo.
Se apenas um ovo estiver disponível e desejamos ter certeza de obter o resultado correto, o experimento pode ser realizado de apenas uma maneira. Solte o ovo da janela do primeiro andar; se sobreviver, jogue-o da janela do segundo andar. Continue subindo até que se quebre. No pior dos casos, esse método pode exigir 36 fezes. Suponha que 2 ovos estejam disponíveis. Qual é o menor número de fezes de ovos que funcionam em todos os casos?
O problema não é realmente encontrar o piso crítico, mas apenas decidir os pisos de onde os ovos devem ser descartados, de forma que o número total de tentativas seja minimizado.
Fonte: Wiki para Programação Dinâmica
Método 1 : Recursão.
Neste artigo, discutiremos uma solução para um problema geral com 'n' ovos e 'k' andares. A solução é tentar deixar cair um ovo de cada andar (de 1 a k) e calcular recursivamente o número mínimo de fezes necessárias no pior caso. O piso que dá o valor mínimo no pior caso vai fazer parte da solução.
Nas soluções a seguir, retornamos o número mínimo de tentativas no pior caso; essas soluções podem ser facilmente modificadas para imprimir também os números dos andares de cada teste.
Significado de um cenário de pior caso: o cenário de pior caso fornece ao usuário a segurança do piso da soleira. Por exemplo- Se temos '1' ovo e 'k' andares, vamos começar a deixar cair o ovo do primeiro andar até que o ovo quebre, suponha que no 'k-ésimo' andar, então o número de tentativas para nos dar uma garantia é 'k' .
1) Subestrutura ótima:
Quando derrubamos um ovo de um andar x, pode haver dois casos (1) O ovo se quebra (2) O ovo não se quebra.
- Se o ovo quebrar depois de cair do 'xº' andar, então só precisamos verificar se há andares abaixo de 'x' com os ovos restantes, pois deve haver algum andar abaixo de 'x' no qual o ovo não quebraria; portanto, o problema se reduz a x-1 andares e n-1 ovos.
- Se o ovo não quebrar depois de cair do 'xº' andar, então só precisamos verificar se há andares mais altos do que 'x'; portanto, o problema se reduz a andares 'k-x' e n ovos.
Como precisamos minimizar o número de tentativas no pior caso, consideramos o máximo de dois casos. Consideramos o máximo de dois casos acima para cada andar e escolhemos o andar que rende o número mínimo de tentativas.
k ==> Número de pisos
n ==> Número de ovos
eggDrop (n, k) ==> Número mínimo de tentativas necessárias para encontrar o
piso crítico no pior caso.
eggDrop (n, k) = 1 + min {max (eggDrop (n - 1, x - 1), eggDrop (n, k - x)), onde x está em {1, 2, ..., k}}
Conceito de pior caso:
Por exemplo:
Haja '2' ovos e '2' andares então-:
Se tentarmos jogar do '1º' andar:
Número de tentativas no pior caso = 1 + max (0, 1)
0 => Se o ovo se quebra do primeiro andar, em seguida, é o piso de soleira (a melhor possibilidade de caso).
1 => Se o ovo não quebrar do primeiro andar, teremos agora '2' ovos e 1 andar para testar que dará a resposta como
'1'. (Pior possibilidade)
Levamos em consideração a possibilidade do pior caso, então 1 + max (0, 1) = 2
Se tentarmos arremessar do '2º' andar:
Número de tentativas no pior caso = 1 + max (1, 0)
1 => Se o ovo quebra do segundo andar, então teremos 1 ovo e 1 andar para encontrar o piso da soleira. (Pior caso)
0 => Se o ovo não quebrar do segundo andar, então é o piso da soleira. (Melhor caso)
Nós consideramos a possibilidade do pior caso para garantia, então 1 + máx (1, 0) = 2.
A resposta final é min (1º, 2º, 3º ..., kº andar)
Portanto, a resposta aqui é '2'.
Abaixo está a implementação da abordagem acima:
#include <bits/stdc++.h>
using namespace std;
// A utility function to get
// maximum of two integers
int max(int a, int b)
{
return (a > b) ? a : b;
}
// Function to get minimum
// number of trials needed in worst
// case with n eggs and k floors
int eggDrop(int n, int k)
{
// If there are no floors,
// then no trials needed.
// OR if there is one floor,
// one trial needed.
if (k == 1 || k == 0)
return k;
// We need k trials for one
// egg and k floors
if (n == 1)
return k;
int min = INT_MAX, x, res;
// Consider all droppings from
// 1st floor to kth floor and
// return the minimum of these
// values plus 1.
for (x = 1; x <= k; x++) {
res = max(
eggDrop(n - 1, x - 1),
eggDrop(n, k - x));
if (res < min)
min = res;
}
return min + 1;
}
// Driver program to test
// to pront printDups
int main()
{
int n = 2, k = 10;
cout << "Minimum number of trials "
"in worst case with "
<< n << " eggs and " << k
<< " floors is "
<< eggDrop(n, k) << endl;
return 0;
}
// This code is contributed
// by Akanksha Rai
#include <limits.h>
#include <stdio.h>
// A utility function to get
// maximum of two integers
int max(int a, int b)
{
return (a > b) ? a : b;
}
/* Function to get minimum number of
trials needed in worst case with n eggs
and k floors */
int eggDrop(int n, int k)
{
// If there are no floors, then no
// trials needed. OR if there is
// one floor, one trial needed.
if (k == 1 || k == 0)
return k;
// We need k trials for one egg and
// k floors
if (n == 1)
return k;
int min = INT_MAX, x, res;
// Consider all droppings from 1st
// floor to kth floor and
// return the minimum of these values
// plus 1.
for (x = 1; x <= k; x++) {
res = max(
eggDrop(n - 1, x - 1),
eggDrop(n, k - x));
if (res < min)
min = res;
}
return min + 1;
}
/* Driver program to test to pront printDups*/
int main()
{
int n = 2, k = 10;
printf("nMinimum number of trials in "
"worst case with %d eggs and "
"%d floors is %d \n",
n, k, eggDrop(n, k));
return 0;
}
public class GFG {
/* Function to get minimum number of
trials needed in worst case with n
eggs and k floors */
static int eggDrop(int n, int k)
{
// If there are no floors, then
// no trials needed. OR if there
// is one floor, one trial needed.
if (k == 1 || k == 0)
return k;
// We need k trials for one egg
// and k floors
if (n == 1)
return k;
int min = Integer.MAX_VALUE;
int x, res;
// Consider all droppings from
// 1st floor to kth floor and
// return the minimum of these
// values plus 1.
for (x = 1; x <= k; x++) {
res = Math.max(eggDrop(n - 1, x - 1),
eggDrop(n, k - x));
if (res < min)
min = res;
}
return min + 1;
}
// Driver code
public static void main(String args[])
{
int n = 2, k = 10;
System.out.print("Minimum number of "
+ "trials in worst case with "
+ n + " eggs and " + k
+ " floors is " + eggDrop(n, k));
}
// This code is contributed by Ryuga.
}
import sys
# Function to get minimum number of trials
# needed in worst case with n eggs and k floors
def eggDrop(n, k):
# If there are no floors, then no trials
# needed. OR if there is one floor, one
# trial needed.
if (k == 1 or k == 0):
return k
# We need k trials for one egg
# and k floors
if (n == 1):
return k
min = sys.maxsize
# Consider all droppings from 1st
# floor to kth floor and return
# the minimum of these values plus 1.
for x in range(1, k + 1):
res = max(eggDrop(n - 1, x - 1),
eggDrop(n, k - x))
if (res < min):
min = res
return min + 1
# Driver Code
if __name__ == "__main__":
n = 2
k = 10
print("Minimum number of trials in worst case with",
n, "eggs and", k, "floors is", eggDrop(n, k))
# This code is contributed by ita_c
using System;
class GFG {
/* Function to get minimum number of
trials needed in worst case with n
eggs and k floors */
static int eggDrop(int n, int k)
{
// If there are no floors, then
// no trials needed. OR if there
// is one floor, one trial needed.
if (k == 1 || k == 0)
return k;
// We need k trials for one egg
// and k floors
if (n == 1)
return k;
int min = int.MaxValue;
int x, res;
// Consider all droppings from
// 1st floor to kth floor and
// return the minimum of these
// values plus 1.
for (x = 1; x <= k; x++) {
res = Math.Max(eggDrop(n - 1, x - 1),
eggDrop(n, k - x));
if (res < min)
min = res;
}
return min + 1;
}
// Driver code
static void Main()
{
int n = 2, k = 10;
Console.Write("Minimum number of "
+ "trials in worst case with "
+ n + " eggs and " + k
+ " floors is " + eggDrop(n, k));
}
}
// This code is contributed by Sam007.
<script>
/* Function to get minimum number of
trials needed in worst case with n
eggs and k floors */
function eggDrop(n,k)
{
// If there are no floors, then
// no trials needed. OR if there
// is one floor, one trial needed.
if (k == 1 || k == 0)
return k;
// We need k trials for one egg
// and k floors
if (n == 1)
return k;
let min = Number.MAX_VALUE;
let x, res;
// Consider all droppings from
// 1st floor to kth floor and
// return the minimum of these
// values plus 1.
for (x = 1; x <= k; x++)
{
res = Math.max(eggDrop(n - 1, x - 1),
eggDrop(n, k - x));
if (res < min)
min = res;
}
return min + 1;
}
// Driver code
let n = 2, k = 10;
document.write("Minimum number of "
+ "trials in worst case with "
+ n + " eggs and " + k
+ " floors is " + eggDrop(n, k));
// This code is contributed by avanitrachhadiya2155
</script>
O número mínimo de tentativas no pior caso com 2 ovos e 10 andares é 4
Saída:
Minimum number of trials in worst case with 2 eggs and 10 floors is 4
Deve-se notar que a função acima calcula os mesmos subproblemas repetidas vezes. Veja a seguinte árvore de recursão parcial, E (2, 2) está sendo avaliada duas vezes. Haverá muitos subproblemas repetidos quando você desenhar a árvore de recursão completa, mesmo para pequenos valores de n e k.
E(2, 4) | ------------------------------------- | | | | | | | | x=1/ x=2/ x=3/ x=4/ / / .... .... / / E(1, 0) E(2, 3) E(1, 1) E(2, 2) / /... / x=1/ ..... / E(1, 0) E(2, 2) / ...... Partial recursion tree for 2 eggs and 4 floors.
Análise de complexidade:
- Complexidade do tempo: Como há um caso de subproblemas sobrepostos, a complexidade do tempo é exponencial.
- Espaço auxiliar: O (1). Como não havia uso de nenhuma estrutura de dados para armazenamento de valores.
Como os mesmos subproblemas são chamados novamente, esse problema possui a propriedade Overlapping Subproblems. Então Egg Dropping Puzzle tem ambas as propriedades (veja isto e isto ) de um problema de programação dinâmica. Como outros problemas típicos de Programação Dinâmica (DP) , recomputações dos mesmos subproblemas podem ser evitadas construindo um array temporário eggFloor [] [] de baixo para cima.
Método 2 : Programação dinâmica.
Nesta abordagem, trabalhamos com a mesma ideia descrita acima, negligenciando o caso de calcular as respostas para os subproblemas repetidas vezes.. A abordagem será fazer uma tabela que armazenará os resultados dos subproblemas de forma que, para resolver um subproblema, seja necessária apenas uma consulta na tabela que levará um tempo constante , que antes demorava um tempo exponencial .
Formalmente, para preencher o DP [i] [j], indique onde 'i' é o número de ovos e 'j' é o número de andares:
- Temos que atravessar para cada andar 'x' de '1' a 'j' e encontrar o mínimo de:
(1 + max( DP[i-1][j-1], DP[i][j-x] )).
Esta simulação deixará as coisas claras:
i => Número de ovos
j => Número de andares
Procure encontrar o máximo
Vamos preencher a tabela para o seguinte caso:
Andares = '4'
Ovos = '2'
1 2 3 4
1 2 3 4 => 1
1 2 2 3 => 2
Para 'ovo-1' cada caso é o caso base, então o
número de tentativas é igual ao número do andar.
Para 'ovo-2', será necessária '1' tentativa para o primeiro
andar, que é o caso básico.
Para o andar 2 =>
Tomando 1º andar 1 + max (0, DP [1] [1])
Tomando 2º andar 1 + max (DP [1] [1], 0)
DP [2] [2] = min ( 1 + max (0, DP [1] [1]), 1 + max (DP [1] [1], 0))
Para o andar-3 =>
Tomando o 1º andar 1 + max (0, DP [2] [ 2])
Tomando o 2º andar 1 + max (DP [1] [1], DP [2] [1])
Tomando 3º andar 1 + max (0, DP [2] [2])
DP [2] [3] = min ('todos os três andares') = 2
Para andar-4 =>
Tomando 1º andar 1 + max (0, DP [2] [3])
Tomando 2 º andar 1 + max (DP [1] [1], DP [2] [2])
Tomando º andar 1 + max (DP [1] [2], DP [2] [1])
Tomando o 4º andar 1 + max (0, DP [2] [3])
DP [2] [4] = min ('todos os quatro andares') = 3
// A Dynamic Programming based for
// the Egg Dropping Puzzle
#include <limits.h>
#include <stdio.h>
// A utility function to get
// maximum of two integers
int max(int a, int b)
{
return (a > b) ? a : b;
}
/* Function to get minimum
number of trials needed in worst
case with n eggs and k floors */
int eggDrop(int n, int k)
{
/* A 2D table where entry
eggFloor[i][j] will represent
minimum number of trials needed for
i eggs and j floors. */
int eggFloor[n + 1][k + 1];
int res;
int i, j, x;
// We need one trial for one floor and 0
// trials for 0 floors
for (i = 1; i <= n; i++) {
eggFloor[i][1] = 1;
eggFloor[i][0] = 0;
}
// We always need j trials for one egg
// and j floors.
for (j = 1; j <= k; j++)
eggFloor[1][j] = j;
// Fill rest of the entries in table using
// optimal substructure property
for (i = 2; i <= n; i++) {
for (j = 2; j <= k; j++) {
eggFloor[i][j] = INT_MAX;
for (x = 1; x <= j; x++) {
res = 1 + max(
eggFloor[i - 1][x - 1],
eggFloor[i][j - x]);
if (res < eggFloor[i][j])
eggFloor[i][j] = res;
}
}
}
// eggFloor[n][k] holds the result
return eggFloor[n][k];
}
/* Driver program to test to pront printDups*/
int main()
{
int n = 2, k = 36;
printf("\nMinimum number of trials "
"in worst case with %d eggs and "
"%d floors is %d \n",
n, k, eggDrop(n, k));
return 0;
}
// A Dynamic Programming based Java
// Program for the Egg Dropping Puzzle
class EggDrop {
// A utility function to get
// maximum of two integers
static int max(int a, int b)
{
return (a > b) ? a : b;
}
/* Function to get minimum number
of trials needed in worst
case with n eggs and k floors */
static int eggDrop(int n, int k)
{
/* A 2D table where entry eggFloor[i][j]
will represent minimum number of trials
needed for i eggs and j floors. */
int eggFloor[][] = new int[n + 1][k + 1];
int res;
int i, j, x;
// We need one trial for one floor and
// 0 trials for 0 floors
for (i = 1; i <= n; i++) {
eggFloor[i][1] = 1;
eggFloor[i][0] = 0;
}
// We always need j trials for one egg
// and j floors.
for (j = 1; j <= k; j++)
eggFloor[1][j] = j;
// Fill rest of the entries in table using
// optimal substructure property
for (i = 2; i <= n; i++) {
for (j = 2; j <= k; j++) {
eggFloor[i][j] = Integer.MAX_VALUE;
for (x = 1; x <= j; x++) {
res = 1 + max(
eggFloor[i - 1][x - 1],
eggFloor[i][j - x]);
if (res < eggFloor[i][j])
eggFloor[i][j] = res;
}
}
}
// eggFloor[n][k] holds the result
return eggFloor[n][k];
}
/* Driver program to test to pront printDups*/
public static void main(String args[])
{
int n = 2, k = 10;
System.out.println("Minimum number of trials in worst"
+ " case with "
+ n + " eggs and "
+ k + " floors is " + eggDrop(n, k));
}
}
/*This code is contributed by Rajat Mishra*/
# A Dynamic Programming based Python Program for the Egg Dropping Puzzle
INT_MAX = 32767
# Function to get minimum number of trials needed in worst
# case with n eggs and k floors
def eggDrop(n, k):
# A 2D table where entry eggFloor[i][j] will represent minimum
# number of trials needed for i eggs and j floors.
eggFloor = [[0 for x in range(k + 1)] for x in range(n + 1)]
# We need one trial for one floor and0 trials for 0 floors
for i in range(1, n + 1):
eggFloor[i][1] = 1
eggFloor[i][0] = 0
# We always need j trials for one egg and j floors.
for j in range(1, k + 1):
eggFloor[1][j] = j
# Fill rest of the entries in table using optimal substructure
# property
for i in range(2, n + 1):
for j in range(2, k + 1):
eggFloor[i][j] = INT_MAX
for x in range(1, j + 1):
res = 1 + max(eggFloor[i-1][x-1], eggFloor[i][j-x])
if res < eggFloor[i][j]:
eggFloor[i][j] = res
# eggFloor[n][k] holds the result
return eggFloor[n][k]
# Driver program to test to pront printDups
n = 2
k = 36
print("Minimum number of trials in worst case with" + str(n) + "eggs and "
+ str(k) + " floors is " + str(eggDrop(n, k)))
# This code is contributed by Bhavya Jain
// A Dynamic Programming based C# Program
// for the Egg Dropping Puzzle
using System;
class GFG {
// A utility function to get maximum of
// two integers
static int max(int a, int b)
{
return (a > b) ? a : b;
}
/* Function to get minimum number of
trials needed in worst case with n
eggs and k floors */
static int eggDrop(int n, int k)
{
/* A 2D table where entry eggFloor[i][j]
will represent minimum number of trials
needed for i eggs and j floors. */
int[, ] eggFloor = new int[n + 1, k + 1];
int res;
int i, j, x;
// We need one trial for one floor and0
// trials for 0 floors
for (i = 1; i <= n; i++) {
eggFloor[i, 1] = 1;
eggFloor[i, 0] = 0;
}
// We always need j trials for one egg
// and j floors.
for (j = 1; j <= k; j++)
eggFloor[1, j] = j;
// Fill rest of the entries in table
// using optimal substructure property
for (i = 2; i <= n; i++) {
for (j = 2; j <= k; j++) {
eggFloor[i, j] = int.MaxValue;
for (x = 1; x <= j; x++) {
res = 1 + max(eggFloor[i - 1, x - 1],
eggFloor[i, j - x]);
if (res < eggFloor[i, j])
eggFloor[i, j] = res;
}
}
}
// eggFloor[n][k] holds the result
return eggFloor[n, k];
}
// Driver function
public static void Main()
{
int n = 2, k = 36;
Console.WriteLine("Minimum number of trials "
+ "in worst case with " + n + " eggs and "
+ k + "floors is " + eggDrop(n, k));
}
}
// This code is contributed by Sam007.
<?php
// A Dynamic Programming based PHP
// Program for the Egg Dropping Puzzle
/* Function to get minimum number
of trials needed in worst
case with n eggs and k floors */
function eggDrop($n, $k)
{
/* A 2D table where entry eggFloor[i][j]
will represent minimum number of
trials needed for i eggs and j floors. */
$eggFloor = array(array());;
// We need one trial for one
// floor and0 trials for 0 floors
for ($i = 1; $i <=$n;$i++)
{
$eggFloor[$i][1] = 1;
$eggFloor[$i][0] = 0;
}
// We always need j trials
// for one egg and j floors.
for ($j = 1; $j <= $k; $j++)
$eggFloor[1][$j] = $j;
// Fill rest of the entries in
// table using optimal substructure
// property
for ($i = 2; $i <= $n; $i++)
{
for ($j = 2; $j <= $k; $j++)
{
$eggFloor[$i][$j] = 999999;
for ($x = 1; $x <= $j; $x++)
{
$res = 1 + max($eggFloor[$i - 1][$x - 1],
$eggFloor[$i][$j - $x]);
if ($res < $eggFloor[$i][$j])
$eggFloor[$i][$j] = $res;
}
}
}
// eggFloor[n][k] holds the result
return $eggFloor[$n][$k];
}
// Driver Code
$n = 2;
$k = 36;
echo "Minimum number of trials in worst case with " .$n. " eggs and "
.$k. " floors is " .eggDrop($n, $k) ;
// This code is contributed by Sam007
?>
<script>
// A Dynamic Programming based Javascript
// Program for the Egg Dropping Puzzle
// A utility function to get
// maximum of two integers
function max(a,b)
{
return (a > b) ? a : b;
}
/* Function to get minimum number
of trials needed in worst
case with n eggs and k floors */
function eggDrop(n,k)
{
/* A 2D table where entry eggFloor[i][j]
will represent minimum number of trials
needed for i eggs and j floors. */
let eggFloor = new Array(n + 1);
for(let i=0;i<(n+1);i++)
{
eggFloor[i]=new Array(k+1);
}
let res;
let i, j, x;
// We need one trial for one floor and
// 0 trials for 0 floors
for (i = 1; i <= n; i++) {
eggFloor[i][1] = 1;
eggFloor[i][0] = 0;
}
// We always need j trials for one egg
// and j floors.
for (j = 1; j <= k; j++)
eggFloor[1][j] = j;
// Fill rest of the entries in table using
// optimal substructure property
for (i = 2; i <= n; i++) {
for (j = 2; j <= k; j++) {
eggFloor[i][j] = Number.MAX_VALUE;
for (x = 1; x <= j; x++) {
res = 1 + max(
eggFloor[i - 1][x - 1],
eggFloor[i][j - x]);
if (res < eggFloor[i][j])
eggFloor[i][j] = res;
}
}
}
// eggFloor[n][k] holds the result
return eggFloor[n][k];
}
/* Driver program to test to pront printDups*/
let n = 2, k = 36;
document.write("Minimum number of trials in worst"
+ " case with "
+ n + " eggs and "
+ k + " floors is " + eggDrop(n, k));
// This code is contributed by ab2127
</script>
O número mínimo de tentativas no pior caso com 2 ovos e 36 andares é 8
Saída :
Minimum number of trials in worst case with 2 eggs and 36 floors is 8
Análise de complexidade:
- Complexidade de tempo: O (n * k ^ 2).
Onde 'n' é o número de ovos e 'k' é o número de andares, já que usamos um loop for aninhado 'k ^ 2' vezes para cada ovo - Espaço auxiliar: O (n * k).
Como um array 2-D de tamanho, 'n * k' é usado para armazenar elementos.
Método 3: Programação dinâmica usando memoization.
#include <bits/stdc++.h>
using namespace std;
#define MAX 1000
vector<vector<int>> memo(MAX, vector<int> (MAX, -1));
int solveEggDrop(int n, int k) {
if(memo[n][k] != -1) { return memo[n][k];}
if (k == 1 || k == 0)
return k;
if (n == 1)
return k;
int min = INT_MAX, x, res;
for (x = 1; x <= k; x++) {
res = max(
solveEggDrop(n - 1, x - 1),
solveEggDrop(n, k - x));
if (res < min)
min = res;
}
memo[n][k] = min+1;
return min + 1;
}
int main() {
int n = 2, k = 36;
cout<<solveEggDrop(n, k);
return 0;
}
// contributed by Shivam Agrawal(shivamagrawal3)
8
Como exercício, você pode tentar modificar a solução DP acima para imprimir todos os andares intermediários (os andares usados para solução de teste mínimo).
Solução mais eficiente : quebra-cabeça de queda de ovos (Coeficiente binomial e solução de pesquisa binária)
Quebra-cabeça de queda de ovo com 2 ovos e andares K
2 ovos e 100 quebra-cabeça de chão
<iframe allow="accelerometer; autoplay; clipboard-write; encrypted-media; gyroscope; picture-in-picture" allowfullscreen="" frameborder="0" height="374" src="https://www.youtube.com/embed/KVfxgpI3Tv0?feature=oembed" width="665"></iframe>
& t = 3s
Referências:
http://archive.ite.journal.informs.org/Vol4No1/Sniedovich/index.php
Escreva comentários se encontrar algo incorreto ou se quiser compartilhar mais informações sobre o tópico discutido acima.
As postagens do blog Acervo Lima te ajudaram? Nos ajude a manter o blog no ar!
Faça uma doação para manter o blog funcionando.
70% das doações são no valor de R$ 5,00...
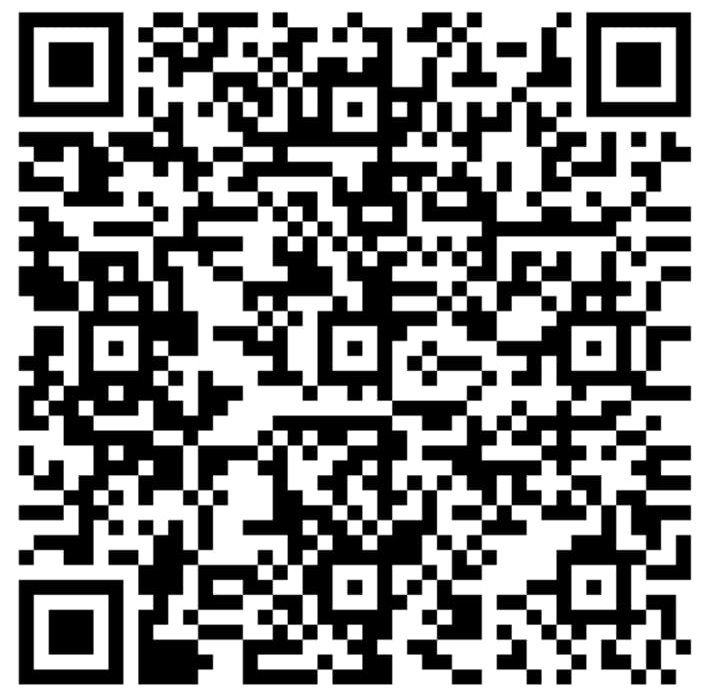
Diógenes Lima da Silva
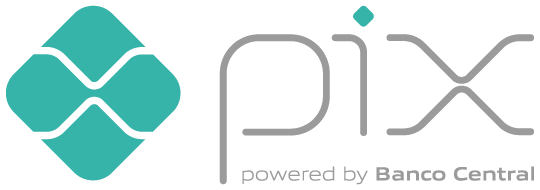