Calculadora de empréstimo usando PyQt5 em Python
Neste artigo, veremos como podemos criar uma calculadora de empréstimo usando PyQt5 . Abaixo está uma imagem que mostra como será a calculadora de empréstimo:
PyQt5 é um kit de ferramentas de interface de usuário de plataforma cruzada, um conjunto de ligações Python para Qt v5. Pode-se desenvolver um aplicativo de desktop interativo com tanta facilidade por causa das ferramentas e simplicidade oferecidas por esta biblioteca
Etapas de implementação da GUI:
1. Crie uma etiqueta de título que exiba o nome da calculadora
2. Crie uma etiqueta e um par de edição de linha para taxa de juros, etiqueta para mostrar o que o usuário deve inserir e edição de linha para inserir o texto
3. Da mesma forma, crie um par para o número de ano e para o valor
4. Crie um botão para calcular
5. Crie um rótulo para mostrar o pagamento mensal calculado
6. Crie um rótulo para mostrar o valor total calculado
Etapas de implementação de back end :
1. Faça a edição de linha para aceitar apenas o número como entrada
2. Adicionar ação ao
botão de ação 3. Dentro da ação de botão de ação obtenha o texto das edições de linha
4. Verifique se o texto de edição de linha está vazio ou zero então retorna para que a função não execute mais
5. Converta o valor do texto em inteiro
6. Calcule o valor mensal e defina este valor para o rótulo
7. Calcule o valor total do valor mensal e mostre este valor através do rótulo
Abaixo está a implementação:
from
PyQt5.QtWidgets
import
*
from
PyQt5
import
QtCore, QtGui
from
PyQt5.QtGui
import
*
from
PyQt5.QtCore
import
*
import
sys
class
Window(QMainWindow):
def
__init__(
self
):
super
().__init__()
self
.setWindowTitle(
"Python "
)
self
.w_width
=
400
self
.w_height
=
500
self
.setGeometry(
100
,
100
,
self
.w_width,
self
.w_height)
self
.UiComponents()
self
.show()
def
UiComponents(
self
):
head
=
QLabel(
"Loan Calculator"
,
self
)
head.setGeometry(
0
,
10
,
400
,
60
)
font
=
QFont(
'Times'
,
15
)
font.setBold(
True
)
font.setItalic(
True
)
font.setUnderline(
True
)
head.setFont(font)
head.setAlignment(Qt.AlignCenter)
color
=
QGraphicsColorizeEffect(
self
)
color.setColor(Qt.darkCyan)
head.setGraphicsEffect(color)
i_label
=
QLabel(
"Annual Interest"
,
self
)
i_label.setAlignment(Qt.AlignCenter)
i_label.setGeometry(
20
,
100
,
170
,
40
)
i_label.setStyleSheet(
"QLabel"
"{"
"border : 2px solid black;"
"background : rgba(70, 70, 70, 35);"
"}"
)
i_label.setFont(QFont(
'Times'
,
9
))
self
.rate
=
QLineEdit(
self
)
onlyInt
=
QIntValidator()
self
.rate.setValidator(onlyInt)
self
.rate.setGeometry(
200
,
100
,
180
,
40
)
self
.rate.setAlignment(Qt.AlignCenter)
self
.rate.setFont(QFont(
'Times'
,
9
))
n_label
=
QLabel(
"Years "
,
self
)
n_label.setAlignment(Qt.AlignCenter)
n_label.setGeometry(
20
,
150
,
170
,
40
)
n_label.setStyleSheet(
"QLabel"
"{"
"border : 2px solid black;"
"background : rgba(70, 70, 70, 35);"
"}"
)
n_label.setFont(QFont(
'Times'
,
9
))
self
.years
=
QLineEdit(
self
)
onlyInt
=
QIntValidator()
self
.years.setValidator(onlyInt)
self
.years.setGeometry(
200
,
150
,
180
,
40
)
self
.years.setAlignment(Qt.AlignCenter)
self
.years.setFont(QFont(
'Times'
,
9
))
a_label
=
QLabel(
"Amount"
,
self
)
a_label.setAlignment(Qt.AlignCenter)
a_label.setGeometry(
20
,
200
,
170
,
40
)
a_label.setStyleSheet(
"QLabel"
"{"
"border : 2px solid black;"
"background : rgba(70, 70, 70, 35);"
"}"
)
a_label.setFont(QFont(
'Times'
,
9
))
self
.amount
=
QLineEdit(
self
)
onlyInt
=
QIntValidator()
self
.amount.setValidator(onlyInt)
self
.amount.setGeometry(
200
,
200
,
180
,
40
)
self
.amount.setAlignment(Qt.AlignCenter)
self
.amount.setFont(QFont(
'Times'
,
9
))
calculate
=
QPushButton(
"Compute Payment"
,
self
)
calculate.setGeometry(
125
,
270
,
150
,
40
)
calculate.clicked.connect(
self
.calculate_action)
self
.m_payment
=
QLabel(
self
)
self
.m_payment.setAlignment(Qt.AlignCenter)
self
.m_payment.setGeometry(
50
,
340
,
300
,
60
)
self
.m_payment.setStyleSheet(
"QLabel"
"{"
"border : 3px solid black;"
"background : white;"
"}"
)
self
.m_payment.setFont(QFont(
'Arial'
,
11
))
self
.y_payment
=
QLabel(
self
)
self
.y_payment.setAlignment(Qt.AlignCenter)
self
.y_payment.setGeometry(
50
,
410
,
300
,
60
)
self
.y_payment.setStyleSheet(
"QLabel"
"{"
"border : 3px solid black;"
"background : white;"
"}"
)
self
.y_payment.setFont(QFont(
'Arial'
,
11
))
def
calculate_action(
self
):
annualInterestRate
=
self
.rate.text()
if
len
(annualInterestRate)
=
=
0
or
annualInterestRate
=
=
'0'
:
return
numberOfYears
=
self
.years.text()
if
len
(numberOfYears)
=
=
0
or
numberOfYears
=
=
'0'
:
return
loanAmount
=
self
.amount.text()
if
len
(loanAmount)
=
=
0
or
loanAmount
=
=
'0'
:
return
annualInterestRate
=
int
(annualInterestRate)
numberOfYears
=
int
(numberOfYears)
loanAmount
=
int
(loanAmount)
monthlyInterestRate
=
annualInterestRate
/
1200
monthlyPayment
=
loanAmount
*
monthlyInterestRate
/
(
1
-
1
/
(
1
+
monthlyInterestRate)
*
*
(numberOfYears
*
12
))
monthlyPayment
=
"{:.2f}"
.
format
(monthlyPayment)
self
.m_payment.setText(
"Monthly Payment : "
+
str
(monthlyPayment))
totalPayment
=
float
(monthlyPayment)
*
12
*
numberOfYears
totalPayment
=
"{:.2f}"
.
format
(totalPayment)
self
.y_payment.setText(
"Total Payment : "
+
str
(totalPayment))
App
=
QApplication(sys.argv)
window
=
Window()
sys.exit(App.
exec
())
Resultado :
As postagens do blog Acervo Lima te ajudaram? Nos ajude a manter o blog no ar!
Faça uma doação para manter o blog funcionando.
70% das doações são no valor de R$ 5,00...
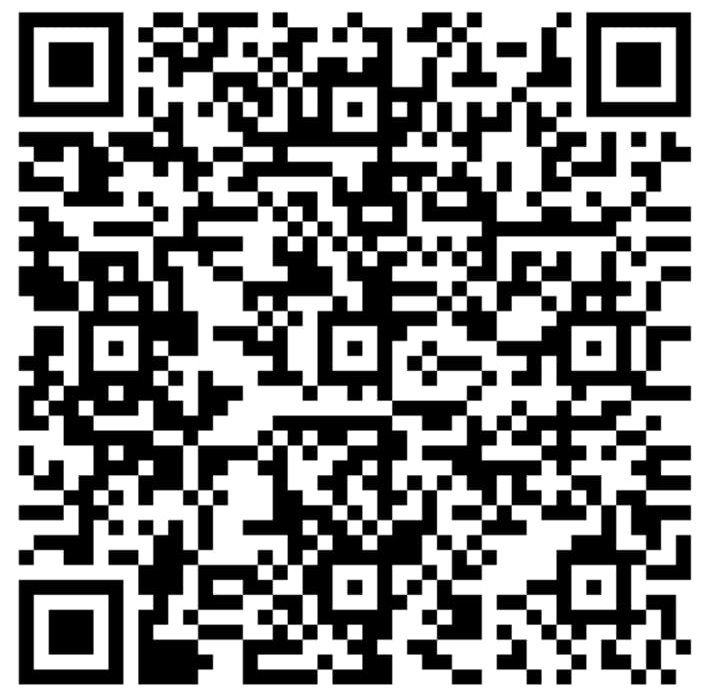
Diógenes Lima da Silva
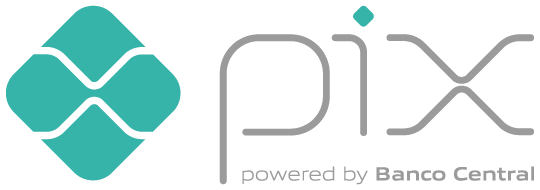