jQuery | Método css()
A biblioteca JQuery suporta quase todos os seletores incluídos na Cascading Style Sheet (CSS). O método css() em JQuery é usado para alterar a propriedade de estilo do elemento selecionado. O css() em JQuery pode ser usado de maneiras diferentes.
O método css() pode ser usado para verificar o valor presente da propriedade para o elemento selecionado:
Sintaxe:
$(selector).css(property)
Valor de retorno: Ele retornará o valor da propriedade para o elemento selecionado.
Exemplo 1:
Input: $("p").css("color"); Output: Output of the above input will return the rgb() value of the element.
Código # 1:
<!DOCTYPE html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs
/jquery/3.3.1/jquery.min.js">
// this is the link of JQuery CDN direct from the
// jquery website so that we can use all the
//function of JQuery css()
</script>
</head>
<body>
<button>Click here and it will return the color value
of p element</button>
<p style="color:green">Wecome to gfg!</p>
</body>
<script>
$(document).ready(function() {
//here selecting button element
$("button").click(function() {
// here when the button is clicked css() method
// will return the value using alert method
alert($("p").css("color"));
});
});
</script>
</html>
Resultado:
antes de clicar no botão-
Depois de clicar no botão-
O método css() também é usado para adicionar ou alterar a propriedade do elemento selecionado.
Sintaxe:
$(selector).css(property, value)
Valor de retorno: isso mudará o valor da propriedade para o elemento selecionado.
Exemplo 2:
Input: $("p").css("color", "red"); Output: Output of the "p" element becomes red whatever may be the color previously.
Código # 2:
<!DOCTYPE html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs
/jquery/3.3.1/jquery.min.js">
// this is the link of JQuery CDN direct from
// the jquery website so that we can use all
// the function of JQuery css()
</script>
</head>
<body>
<button>Click here and it will return the color value
of p element</button>
<p style="border: 2px solid green;color:red;padding:5px">
Wecome to gfg!.</p>
</body>
<script>
$(document).ready(function() {
// here selecting button element
$("button").click(function() {
// here when the button is clicked css()
// method will change the color of paragraph
$("p").css("color", "green");
});
});
</script>
</html>
Resultado:
antes de clicar no botão-
Depois de clicar no botão-
O método css() pode usar a função para alterar a propriedade css do elemento selecionado:
Sintaxe:
$(selector).css(property, function(index, currentvalue))
Valor de retorno: Ele retornará com o valor alterado para a propriedade selecionada.
Exemplo 3:
Input: $("p").css("padding", function(i){ return i+20;}); Output: Output will get is the paragraph with padding value increases to "25px" whatever be the initial value.
Código # 3:
<!DOCTYPE html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs
/jquery/3.3.1/jquery.min.js">
//this is the link of JQuery CDN direct from
//the jquery website so that we can use all
//the function of JQuery css()
</script>
</head>
<body>
<button>Click here and the padding will change.</button>
<p style="border: 2px solid green;color:green;padding=5px;">
Welcome to gfg!.</p>
</body>
<script>
$(document).ready(function() {
$("button").click(function() {
$("p").css("padding", function(h) {
return h + 30;
});
});
});
</script>
</html>
Resultado:
antes de clicar no botão-
Depois de clicar no botão-
Também podemos aplicar mais de uma propriedade por vez em JQuery com a ajuda do método css.
Observação: neste método, escrevemos o nome da propriedade em camelCase.
Sintaxe:
$(selector).css({property:value, property:value, ...})
Código # 4:
<!DOCTYPE html>
<html>
<head>
<title>Document</title>
</head>
<body>
<p style="border: 2px solid green;color:green;padding=5px;">
Welcome to gfg!.</p>
<button>Apply css</button>
<script src=
"https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js">
</script>
<script>
$("button").click(function(){
//applying more than one property at a time
//Note : property name written in camelCase
$("p").css({"backgroundColor":"green",
"color":"white","fontSize":"20px"});
});
</script>
</body>
</html>
Saída:
Antes de clicar no botão-
Depois de clicar no botão-
As postagens do blog Acervo Lima te ajudaram? Nos ajude a manter o blog no ar!
Faça uma doação para manter o blog funcionando.
70% das doações são no valor de R$ 5,00...
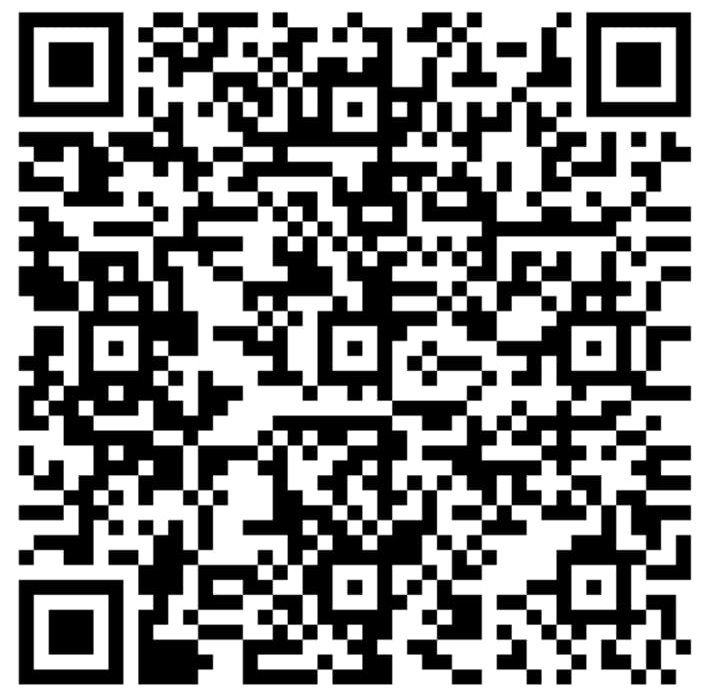
Diógenes Lima da Silva
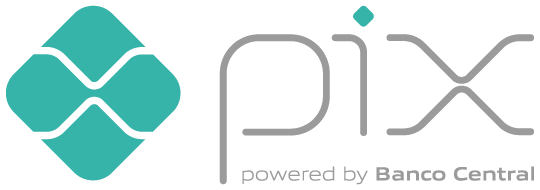